Authorization
Before you begin, you'll need an API key. Sign up for an account here, then make a project, then create an API key.
Understanding Auth
Room Service uses your own authentication/authorization. We ask you to setup an "Auth Webhook" that accepts POST
requests on your own server.
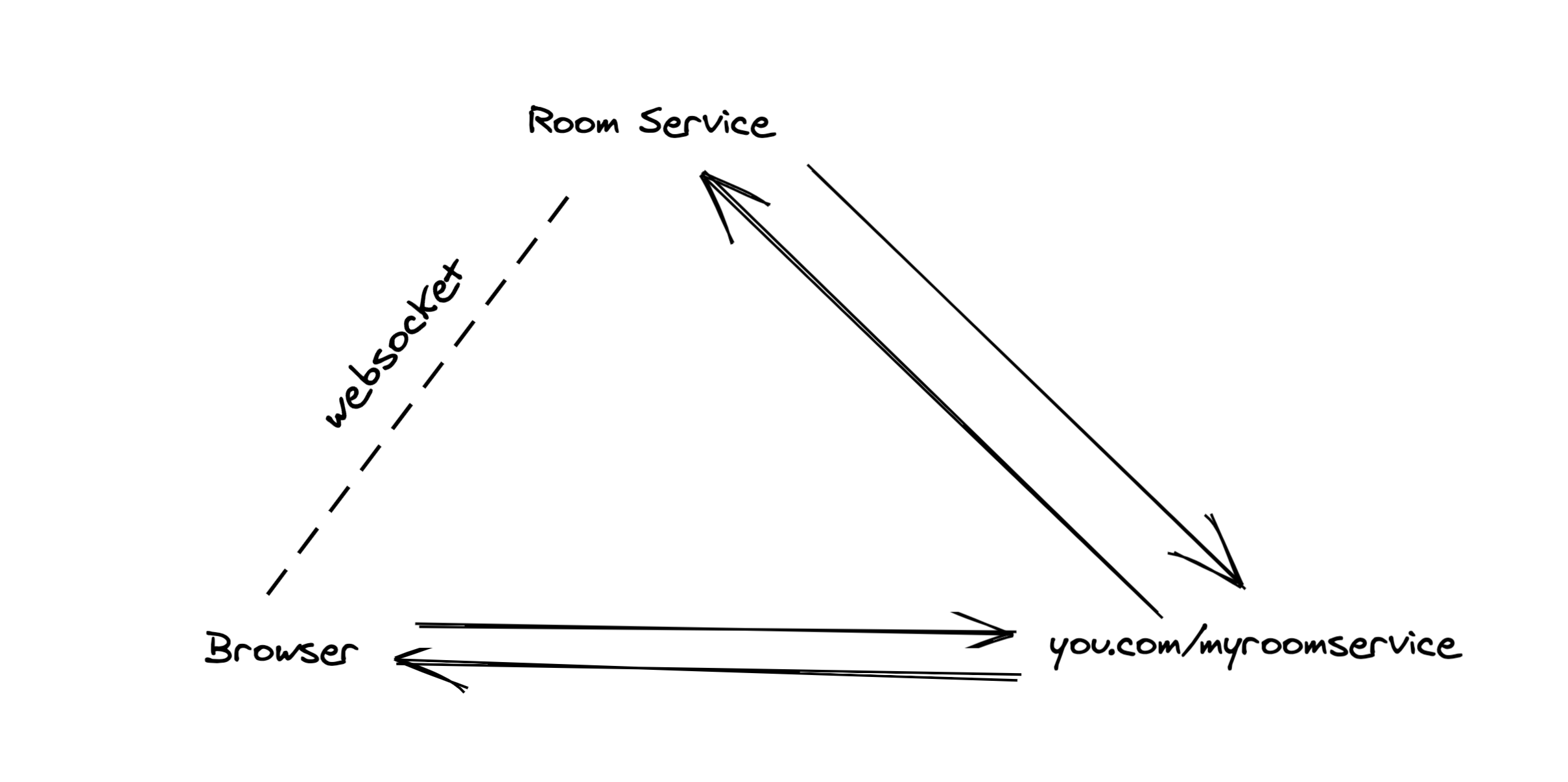
The browser client will send your endpoint a POST
body that contains the resources it would like to access:
1POST /your_webhook2{3 resources: [4 {5 object: 'room',6 reference: myRoomName,7 permission: 'join',8 },9 ],10}
At the moment, a document
gives full access to all maps and lists. In the future, we'll have more granular access control.
If you decide a user that this request is coming from someone who's not logged in, just return a 401.
1// Node.js example2function handleRoomService(req, res) {3 if (!isLoggedIn(req)) {4 return res.send(401)5 }67 // ...8}
If you want to authorize this user, then forward the body you got from the browser via a POST request to super.roomservice.dev/provision along with your API key, and an id to represent this user.
1const user = "this-user-id"2const body = req.body34const r = await fetch('https://super.roomservice.dev/provision', {5 method: 'post',6 headers: {7 Authorization: `Bearer: ${___APIKEY___}`,8 'Content-Type': 'application/json',9 },10 body: JSON.stringify({11 user: user,12 resources: body,13 }),14})
Finally, return the body received from Room Service back to the client:
1res.json(await r.json())
Full example in Node.js / express:
1// server.js23const express = require("express");4const fetch = require("node-fetch");5const app = express();6const port = 3002;7app.use(express.json());89// Replace this with your authorization scheme.10function isLoggedIn(req) {11 return true; // for the moment, we'll just let everyone in12}1314app.post("/my-roomservice", async (req, res) => {15 if (!isLoggedIn(req)) {16 return res.send(401);17 }1819 // In practice, this should be whatever user id YOU use.20 const user = Math.random().toString(36).substr(2, 9);21 const body = req.body;2223 const r = await fetch("https://super.roomservice.dev/provision", {24 method: "post",25 headers: {26 Authorization: `Bearer: ${___APIKEY___}`,27 "Content-Type": "application/json",28 },29 body: JSON.stringify({30 user: user,31 resources: body.resources,32 }),33 });3435 return res.json(await r.json());36});3738app.listen(port, () => {39 console.log(`Example app listening at http://localhost:${port}`);40});41